Quantum Katas #2: Superposition
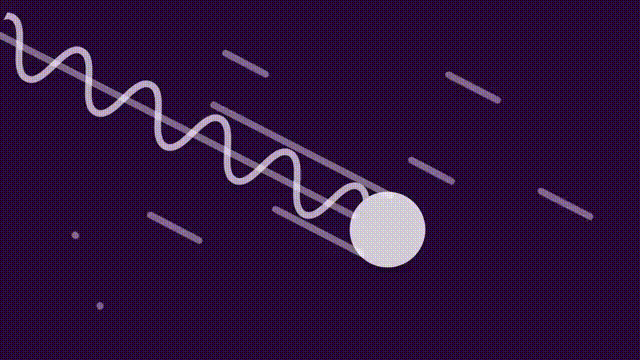
Intro⌗
If you read my last guide on basic quantum gates, you are familiar with the concept of superposition.
If not, let me briefly explain. A classical computer calculates on a system of binary "bits" that can either occupy the state 0 or 1, on or off.
A quantum computer on the other hand, uses qubits, or quantum bits which can occupy infinitely many states in between 0 and 1. When a qubit is in one of these "hybrid" states, we say that it is in superposition
.
Consider the Bloch sphere below, which provides a visual reference for the state of a qubit.
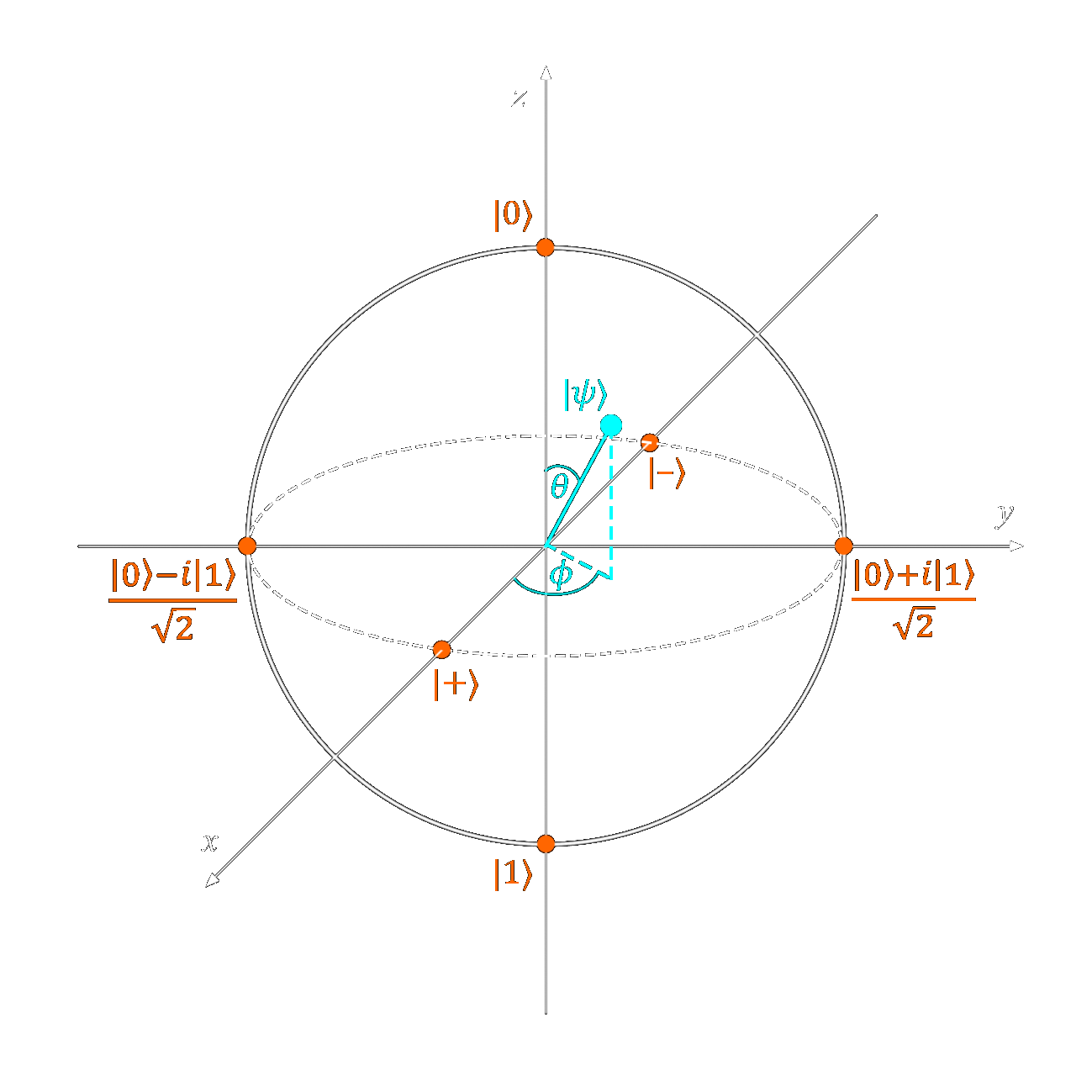
Like a classical bit, the qubit can take states 0 and 1, which are usually referred to as |0⟩ and |1⟩ when describing qubits.
We also see states |+⟩ and |−⟩, which represent a superposition halfway in between |0⟩ and |1⟩.
Finally we see the state vector |Ψ⟩, which can point to an arbitrary state on the surface of the bloch sphere. These arbitrary states are represented by angles θ and ϕ.
The following programming challenges will have you manipulate qubits into various states of superposition. If you have not set up a Q# development environment, I suggest you take a look at my last post.
Task 1: Plus state⌗
As input, we are given a qubit in the |0⟩ state, and we need to output a qubit in the |+⟩ state.
Recall that the Hadamard
gate maps qubits like so:
|0⟩→|+⟩ |1⟩→|−⟩
Since we are only given qubits in the |0⟩ state, we can convert them to |+⟩ by simply applying the H()
operation.
Task 2: Minus state⌗
Like the previous task, we are given a qubit in the |0⟩ state, but now we must map it to the |−⟩ state.
Looking at the mappings for the Hadamard
gate, we can see that applying H()
to a qubit in the |1⟩ state will result in the |−⟩ state. We need to make use of the Pauli X
gate to turn our |0⟩ states into |1⟩.
Task 3: Unequal superposition⌗
As I mentioned in the intro, the angles ϕ and θ on the Bloch sphere translate into an arbitrary superposition.
Let us consider the fact that we are trying to change the probability state of the qubit, which is represented by angle θ between the |1⟩ state and the state vector |Ψ⟩.
Here we are tasked with putting the qubit into a superposition given by the angle α. A hint given in the comments reads:
Experiment with rotation gates from the Microsoft.Quantum.Intrinsic namespace.
Since we know that the probability state can be changed by rotating our state vector about the Y axis, we can leverage the Ry()
operation.
Task 4: Superposition of all basis vectors on two qubits⌗
We finally reached our first 2-qubit gate of this kata.
Our goal is to take two qubits in state |00⟩ and convert them into the state
|00⟩+|01⟩+|10⟩+|11⟩2
Which is an equal probability of all possible outcomes of measurement.
This state is also represented by the tensor product of the two states
|+⟩⊗|+⟩
Since this superposition of qubits is represented by the tensor product of the qubits, we can simply use the H()
gate to change both our qubits to the |+⟩ state.
Task 5: Superposition of basis vectors with phases⌗
Our goal in this task is to take two qubits in the |00⟩ state and convert them to the state
|00⟩+i|01⟩−|10⟩−i|11⟩2
This is another example of a n qubit state that can be broken down into the tensor product of n discreet states. In this case, the state above can be rewritten as
|0⟩−|1⟩√2⊗|0⟩+i|1⟩√2=|−⟩⊗|i⟩
We already know how to put our qubits in these states! Take another look at the Bloch sphere to orient yourself. We are aiming for the |−⟩ and |i⟩ states, which both lie along the XY plane.
We can use the Hadamard
gate to move our state vector to the |+⟩ state, and then use various rotations to get to the final states.
Task 6: Bell state⌗
Again, we are getting an input of two qubits |00⟩. Our task is to create the bell state |Φ+⟩.
If you need a refresher on Bell states, please refer to my last post, where I explain the concept in more detail.
For now, we can simply recall that the |Φ+⟩ can be prepared by giving |00⟩ as an input to the Bell preparation circuit β.
|β00⟩→|Φ+⟩
Since we are already given the qubits in state |00⟩, we can easily prepare the desired bell state.
Task 7: All Bell states⌗
Here the input is the same as the last few tasks. This time however, we are also given an index
, that corresponds to one of the four Bell states.
0:|Φ+⟩=|00⟩+|11⟩√2 1:|Φ−⟩=|00⟩−|11⟩√2 2:|Ψ+⟩=|01⟩+|10⟩√2 3:|Ψ−⟩=|01⟩−|10⟩√2
We are given an input of |00⟩, so unless the input is 0, we will have to alter our qubits after preparing a bell state.
First let's consider the sign of the Bell state. If the index supplied is 1 or 3, it means we are preparing |Ψ−⟩ or |Φ−⟩. Since our input |00⟩→|Φ+⟩, we need to flip the sign.
If you remember task 1.7 from my last post, this can be accomplished with the Z()
operation.
The only thing left to check for now is if we need to go from |Φ±⟩ to |Ψ±⟩
Fill in the operation like so to complete the task.
Task 8: Greenberger–Horne–Zeilinger state⌗
The Greenberger–Horne–Zeilinger state, or GHZ state, is a special, maximally entangled case of 3 or more qubits.
It takes the following form:
GHZn=n⏞|0...0⟩+n⏞|1...1⟩√2
For example, a three qubit GHZ state is as follows
GHZ3=|000⟩+|111⟩√2
The observant may notice the similarity to the two qubit Bell state |Φ+⟩. In fact, the GHZ state can be prepared in a similar way.
Instead of preforming a Controlled NOT
with the only two qubits, we can use the first qubit as the control and CNOT
every additional qubit with it.
In Q#
, you can use the Rest()
operation, which creates a copy of the input list removing the first element. This is the method shown in the solution, but you will have to add
to the top of your Tasks.qs
file.
Task 9: Superposition of all basis vectors⌗
In this task, we need to create an equal superposition of n vectors. That will look like
2n permutations⏞|0...0⟩+...+|1...1⟩√2n
For example:
n=3→|000⟩+|001⟩+|010⟩+|011⟩+|100⟩+|101⟩+|110⟩+|111⟩√23
This can be achieved by simply applying the Hadamard
gate to every qubit. This is the same process we did for task 1.4, except with n qubits.
Task 10. |00⟩ + |01⟩ + |10⟩ state⌗
Here we are tasked with putting two qubits into the state
|Ψ⟩=|00⟩+|01⟩+|10⟩√3
This means our 2 qubit state has a 13 chance of collapsing into each of the states |00⟩,|01⟩,|10⟩, with no chance of being in the state |11⟩
I recommend taking a look at the stack exchange solution for more detail, but I will explain briefly here.
The first qubit in our input can be considered a control bit. For example, if our input is |10⟩, no action is needed and the state will measure |10⟩.
If the first qubit is |0⟩, we need to put our second qubit into the |+⟩ state, which will give an equal probability of measuring |00⟩ and |01⟩.
Now we can think of our qubit in the following form:
|Ψ⟩=√2√3|0⟩|+⟩+1√3|1⟩|0⟩
Which gives it a 66% chance of giving the plus state (which covers |01⟩ and |00⟩) and a 33% chance of going straight to |10⟩. This neatly gives us our 13 chance of measuring any of the states.
How do we replicate this probability with our qubits? Well we want our first qubit to be in the |0⟩ state 23 of the time, so we have to adjust our probability amplitude.
Then for our conditional logic, we can use a "reverse controlled Hadamard", which will put the second qubit in the |+⟩ state if the first qubit is 0.
Task 11: Superposition of |0...0⟩ and given bit string⌗
In this task, we must create a superposition of |0...0⟩ and an input given by a bit string.
The bit string:
- Has the same length as the qubit register
- Is guaranteed to start with a
1
For example, if the bit string was [true, false]
, the state we want to create would be
|00⟩+|10⟩√2
Here is a circuit that illustrates this on the input [true, false, true]
.
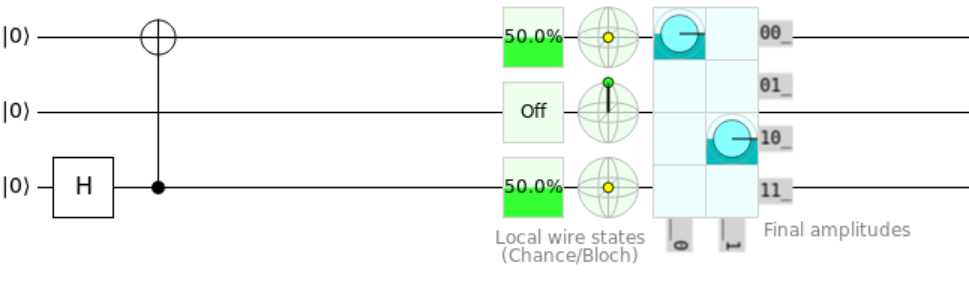
We apply the Hadamard
gate to the first qubit, starting the superposition. For each following qubit, the CNOT
gate is applied if the corresponding entry in the bit string is true
.
The first qubit has a 50% chance of being |0⟩ or |1⟩. If it is zero, no more operations are applied, so the state |000⟩ is measured.
If the state of the first qubit (qs[0]
) is one, all possible states that start with |0..⟩ are no longer possible.
In our above example, the second bit was false, meaning it will never get a NOT
and will remain |0⟩. Hence, any state |.1.⟩ with 1
in the middle are not possible.
Similarly, a measurement of |100⟩ would be impossible since if the first qubit was |1⟩, the third qubit would have the NOT
gate applied.
After eliminating the impossible measurements, the probability matrix gives us an equally probable output of |000⟩ and |101⟩.
Here I used the Length()
function in a ranged for
loop to check the value of the ith bit in the input and apply CNOT
accordingly.
Task 12: Superposition of two bit strings⌗
In this task, we are given two arbitrary bit strings of length n. For example if we had two input strings [false, true, false]
and [false, false, true]
, we would create an equal superposition of like this:
|010⟩+|001⟩√2
The first step in completing this task is to find the spot where the two bitstrings "diverge".
By putting this bit into superposition, we splits our state into two distinct possible outcomes. Here is a helper function that returns the index of the first differing qubit:
Once we found where the strings diverge, we can apply the H()
gate to it to create superposition. Next, we have to iterate through our bitstrings and put the qubits in their final state.
If the bit in both strings at index i are the same, we have two possibilities:
- They are both
false
, meaning no action is needed. - They are both
true
, and we need toNOT
our qubit register at index i
If the bit in both strings at index i are different, we use the first different bit as a control, and CNOT
the qubit at index i.
If the first differing qubit measures as |1⟩, then the ith qubit is flipped to |1⟩.
Then, if the first bitstring at index i is not equal to the value of the first different bit from the first string, we NOT
the qubit at index i.
The pseudocode above can be realized with the following operation:
Task 13: Superposition of four bit strings⌗
This task is like the one above, except we must deal with 4 bitstrings, with N elements each.
Elements can be accessed like bits[i][j]
where 0<i<3 and 0<j<N−1.
First we can use the ApplyToEach()
function to apply the Hadamard
gate to each of the ancilla qubits to put them in the |+⟩ state.
Then we iterate through the rows and columns of our 2D array, touching each qubit. If bits[i][j]
is true, we preform the following operation: (ControlledOnInt(i, X))(anc, qs[j]);
Then we have to iterate through each bitstring. If we are on bits[i]
and i is odd, we do
(ControlledOnBitString(bits[i], X))(qs, anc[0]);
If it's even, we do
(ControlledOnBitString(bits[i], X))(qs, anc[1]);
Here is the implementation.
Task 14: W state on 2ᵏ qubits⌗
A W state is a state of N qubits that takes the following form:
|WN⟩=N states⏞|100...0⟩+|010...0⟩+...+|00..01⟩√N
In this task, we are challenged with creating the state |WN⟩ where N=2k.
We will implement a recursive operation to create the W state.
In this operation, our base case is when N=1, where the W state is trivially prepared. If we are in this state, we can apply X(qs[0])
and return.
If not, we must preform the following steps:
- Create new variable K=N2
- Recursively call the function passing it
qs[0 .. K - 1]
as input - Create an ancilla qubit and apply
H()
to it - For every qubit from 0 to K - 1, do a controlled swap using the ancilla,
qs[i]
, andqs[i + K]
- For every qubit from 0 to N - 1, preform a
CNOT
withqs[i]
and the ancilla.
Task 15: W state on arbitrary number of qubits⌗
Our task here is the same as in the previous, except now we cannot assume that N=2k.
Again, we find the length of are qubit register using the Length()
function.
Our base case for this recursive function is when N=1, where we can again preform X(qs[0])
.
If we aren't at the base case, we want to preform a rotation on the first qubit to put it into the following state:
√N−1N|0⟩+1√N|1⟩
We can accomplish this with an Ry()
operation on the first qubit, rotating by 2θ, where
θ=sin−1(1√N)
Then we preform a zero controlled recursive call of the same function. A zero controlled gate can be preformed by applying a standard controlled gate to a qubit who's state has been flipped.
This recursive loop will continue until we are at the base state, and where we will preform X(qs[0])
and reach our target state.
Conclusion⌗
In this kata, we learned about the various special states and properties of quantum superposition, as well as some nontrivial implementations of these states with Q#.
As always, feel free to reach out with questions or feedback 😄